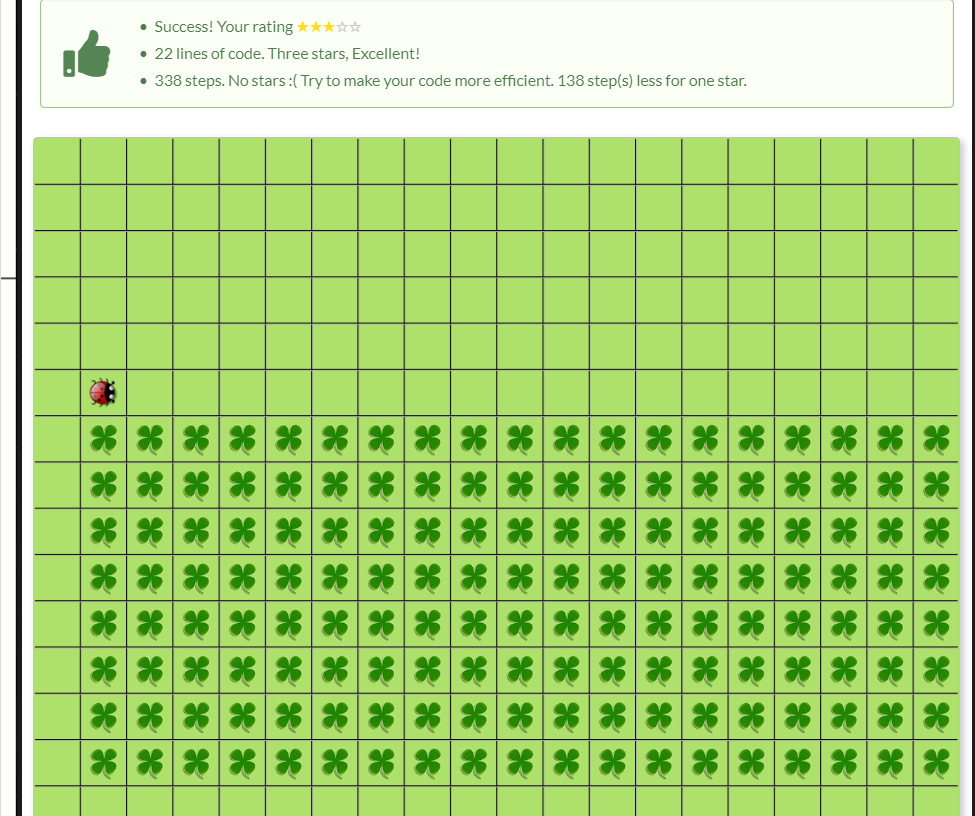
class MyClara extends Clara {
/**
* In the ‘run()’ method, you can write your program for Clara
*/
void run() {
// Get the dimensions of the cake from the user
int cakeWidth = readInt(“Please enter the width of the cake:”);
int cakeHeight = readInt(“Please enter the height of the cake:”);
// Start building the cake layers
while (cakeHeight > 0) {
// Build one row of the cake
for (int i = 0; i < cakeWidth; i++) {
placeCakeBlock();
move();
}
// Move Clara to the next row on the left side
moveLeftToNextRow();
cakeHeight–;
// Build another row of the cake
for (int i = 0; i < cakeWidth; i++) {
placeCakeBlock();
move();
}
// Move Clara to the next row on the right side
moveRightToNextRow();
cakeHeight–;
}
}
// Place a leaf to represent a block of the cake
void placeCakeBlock() {
if (!onLeaf()) {
putLeaf();